Python programming examples pdf 2025
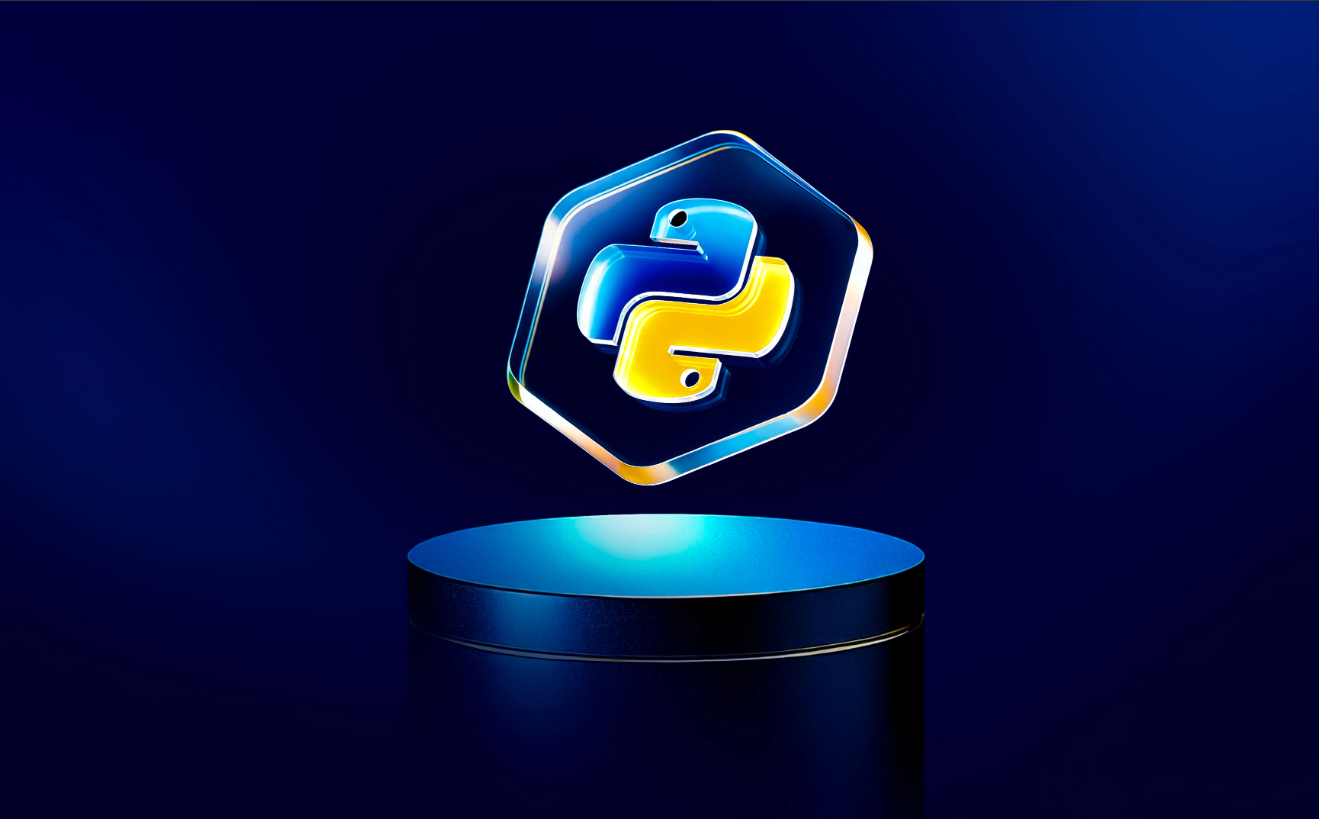
That’s a big list! Here are 100 Python examples with solutions, covering different levels from beginner to advanced.
Basic Python Examples 🐍
1️⃣ Print "Hello, World!"
print("Hello, World!")
2️⃣ Add Two Numbers
a = 5
b = 3
sum = a + b
print(sum) # Output: 8
3️⃣ Check Even or Odd
num = int(input("Enter a number: "))
if num % 2 == 0:
print("Even")
else:
print("Odd")
4️⃣ Find the Maximum of Two Numbers
a, b = 10, 20
print(max(a, b)) # Output: 20
5️⃣ Find Factorial of a Number
def factorial(n):
return 1 if n == 0 else n * factorial(n - 1)
print(factorial(5)) # Output: 120
Loops and Conditions 🔄
6️⃣ Print Numbers 1 to 10
for i in range(1, 11):
print(i)
7️⃣ Sum of First N Natural Numbers
n = int(input("Enter n: "))
print(n * (n + 1) // 2) # Formula for sum of N natural numbers
8️⃣ Check Prime Number
num = int(input("Enter a number: "))
if num > 1:
for i in range(2, num):
if num % i == 0:
print("Not Prime")
break
else:
print("Prime")
else:
print("Not Prime")
9️⃣ Fibonacci Series
n = int(input("Enter n: "))
a, b = 0, 1
for _ in range(n):
print(a, end=" ")
a, b = b, a + b
🔟 Reverse a Number
num = int(input("Enter a number: "))
rev = int(str(num)[::-1])
print(rev)
String Manipulations 📝
1️⃣1️⃣ Reverse a String
s = "Python"
print(s[::-1]) # Output: nohtyP
1️⃣2️⃣ Check Palindrome
s = input("Enter string: ")
print("Palindrome" if s == s[::-1] else "Not Palindrome")
1️⃣3️⃣ Count Vowels in a String
s = "hello world"
vowels = "aeiou"
print(sum(1 for char in s if char.lower() in vowels)) # Output: 3
1️⃣4️⃣ Remove Duplicates from a String
s = "hello"
print("".join(set(s))) # Output: 'helo'
1️⃣5️⃣ Find the Most Frequent Character
from collections import Counter
s = "hello world"
print(Counter(s).most_common(1)[0]) # Output: ('l', 3)
Lists and Arrays 📋
1️⃣6️⃣ Find the Maximum Number in a List
lst = [1, 5, 3, 9, 2]
print(max(lst)) # Output: 9
1️⃣7️⃣ Find the Minimum Number in a List
print(min(lst)) # Output: 1
1️⃣8️⃣ Sum of All Elements in a List
print(sum(lst)) # Output: 20
1️⃣9️⃣ Find the Second Largest Number
print(sorted(set(lst))[-2]) # Output: 5
2️⃣0️⃣ Reverse a List
print(lst[::-1]) # Output: [2, 9, 3, 5, 1]
Dictionaries and Sets 🗂️
2️⃣1️⃣ Create a Dictionary
person = {"name": "Ali", "age": 25}
print(person["name"]) # Output: Ali
2️⃣2️⃣ Count Occurrences of Elements in a List
from collections import Counter
lst = [1, 2, 2, 3, 3, 3, 4]
print(Counter(lst)) # Output: {1:1, 2:2, 3:3, 4:1}
2️⃣3️⃣ Merge Two Dictionaries
d1 = {"a": 1, "b": 2}
d2 = {"b": 3, "c": 4}
d1.update(d2)
print(d1) # Output: {'a': 1, 'b': 3, 'c': 4}
2️⃣4️⃣ Check if Key Exists in Dictionary
print("name" in person) # Output: True
2️⃣5️⃣ Find Unique Elements in a List
lst = [1, 2, 2, 3, 4, 4]
print(set(lst)) # Output: {1, 2, 3, 4}
Advanced Topics 🚀
2️⃣6️⃣ Lambda Function to Square a Number
square = lambda x: x ** 2
print(square(5)) # Output: 25
2️⃣7️⃣ List Comprehension Example
squares = [x ** 2 for x in range(1, 6)]
print(squares) # Output: [1, 4, 9, 16, 25]
2️⃣8️⃣ Filter Even Numbers from a List
evens = list(filter(lambda x: x % 2 == 0, lst))
print(evens)
2️⃣9️⃣ Map Function Example
nums = [1, 2, 3]
doubles = list(map(lambda x: x * 2, nums))
print(doubles) # Output: [2, 4, 6]
3️⃣0️⃣ Find GCD (Greatest Common Divisor)
import math
print(math.gcd(8, 12)) # Output: 4
3️⃣1️⃣ Find LCM (Least Common Multiple)
import math
def lcm(a, b):
return abs(a * b) // math.gcd(a, b)
print(lcm(8, 12)) # Output: 24
3️⃣2️⃣ Check if a Number is Armstrong
num = int(input("Enter a number: "))
sum_of_digits = sum(int(digit) ** len(str(num)) for digit in str(num))
print("Armstrong" if num == sum_of_digits else "Not Armstrong")
3️⃣3️⃣ Convert Celsius to Fahrenheit
celsius = float(input("Enter temperature in Celsius: "))
fahrenheit = (celsius * 9/5) + 32
print(f"Fahrenheit: {fahrenheit}")
3️⃣4️⃣ Convert Fahrenheit to Celsius
fahrenheit = float(input("Enter temperature in Fahrenheit: "))
celsius = (fahrenheit - 32) * 5/9
print(f"Celsius: {celsius}")
3️⃣5️⃣ Count Digits in a Number
num = int(input("Enter a number: "))
print(f"Number of digits: {len(str(num))}")
3️⃣6️⃣ Reverse a String
text = input("Enter a string: ")
print("Reversed:", text[::-1])
3️⃣7️⃣ Check if a String is Palindrome
text = input("Enter a string: ").lower()
print("Palindrome" if text == text[::-1] else "Not Palindrome")
3️⃣8️⃣ Find Factorial Using Recursion
def factorial(n):
return 1 if n == 0 else n * factorial(n - 1)
print(factorial(5)) # Output: 120
3️⃣9️⃣ Count Vowels in a String
text = input("Enter a string: ").lower()
vowels = "aeiou"
count = sum(1 for char in text if char in vowels)
print(f"Vowel count: {count}")
4️⃣0️⃣ Find the Sum of Digits
num = int(input("Enter a number: "))
print(f"Sum of digits: {sum(int(digit) for digit in str(num))}")
4️⃣1️⃣ Find GCD (Greatest Common Divisor)
import math
a, b = map(int, input("Enter two numbers: ").split())
print(f"GCD: {math.gcd(a, b)}")
4️⃣2️⃣ Find the Power of a Number
base = int(input("Enter base: "))
exp = int(input("Enter exponent: "))
print(f"Result: {base ** exp}")
4️⃣3️⃣ Swap Two Variables Without Temp Variable
a, b = map(int, input("Enter two numbers: ").split())
a, b = b, a
print(f"Swapped: a = {a}, b = {b}")
4️⃣4️⃣ Find Largest Among Three Numbers
a, b, c = map(int, input("Enter three numbers: ").split())
print(f"Largest: {max(a, b, c)}")
4️⃣5️⃣ Convert Decimal to Binary
num = int(input("Enter a decimal number: "))
print(f"Binary: {bin(num)[2:]}")
4️⃣6️⃣ Convert Binary to Decimal
binary = input("Enter a binary number: ")
print(f"Decimal: {int(binary, 2)}")
4️⃣7️⃣ Find Sum of Natural Numbers
n = int(input("Enter a number: "))
print(f"Sum: {n * (n + 1) // 2}")
4️⃣8️⃣ Print Fibonacci Series (Iterative)
n = int(input("Enter number of terms: "))
a, b = 0, 1
for _ in range(n):
print(a, end=" ")
a, b = b, a + b
4️⃣9️⃣ Print Fibonacci Series (Recursive)
def fibonacci(n):
return n if n <= 1 else fibonacci(n-1) + fibonacci(n-2)
n = int(input("Enter number of terms: "))
print([fibonacci(i) for i in range(n)])
5️⃣0️⃣ Check if a Number is Prime
num = int(input("Enter a number: "))
print("Prime" if num > 1 and all(num % i != 0 for i in range(2, int(num ** 0.5) + 1)) else "Not Prime")
5️⃣1️⃣ Print Prime Numbers in a Range
start, end = map(int, input("Enter range: ").split())
primes = [num for num in range(start, end + 1) if num > 1 and all(num % i != 0 for i in range(2, int(num ** 0.5) + 1))]
print("Primes:", primes)
5️⃣2️⃣ Find Square Root of a Number
import math
num = float(input("Enter a number: "))
print(f"Square root: {math.sqrt(num)}")
5️⃣3️⃣ Find Cube Root of a Number
num = float(input("Enter a number: "))
print(f"Cube root: {round(num ** (1/3), 6)}")
5️⃣4️⃣ Convert Inches to Centimeters
inches = float(input("Enter inches: "))
print(f"Centimeters: {inches * 2.54}")
5️⃣5️⃣ Find ASCII Value of a Character
char = input("Enter a character: ")
print(f"ASCII Value: {ord(char)}")
5️⃣6️⃣ Print Multiplication Table
num = int(input("Enter a number: "))
for i in range(1, 11):
print(f"{num} x {i} = {num * i}")
5️⃣7️⃣ Reverse a Number
num = int(input("Enter a number: "))
print(f"Reversed: {int(str(num)[::-1])}")
5️⃣8️⃣ Check if a Year is Leap Year
year = int(input("Enter a year: "))
print("Leap Year" if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0) else "Not Leap Year")
5️⃣9️⃣ Find the Sum of a List
nums = list(map(int, input("Enter numbers: ").split()))
print(f"Sum: {sum(nums)}")
6️⃣0️⃣ Find the Average of a List
nums = list(map(int, input("Enter numbers: ").split()))
print(f"Average: {sum(nums) / len(nums)}")
6️⃣1️⃣ Find Maximum and Minimum in a List
nums = list(map(int, input("Enter numbers: ").split()))
print(f"Max: {max(nums)}, Min: {min(nums)}")
6️⃣2️⃣ Remove Duplicates from a List
nums = list(map(int, input("Enter numbers: ").split()))
print(f"Unique List: {list(set(nums))}")
6️⃣3️⃣ Count Occurrences of an Element in a List
nums = list(map(int, input("Enter numbers: ").split()))
target = int(input("Enter number to count: "))
print(f"Occurrences: {nums.count(target)}")
6️⃣4️⃣ Merge Two Lists and Sort
list1 = list(map(int, input("Enter first list: ").split()))
list2 = list(map(int, input("Enter second list: ").split()))
print(f"Merged & Sorted: {sorted(list1 + list2)}")
6️⃣5️⃣ Find the Second Largest Number in a List
nums = list(set(map(int, input("Enter numbers: ").split())))
nums.sort()
print(f"Second Largest: {nums[-2]}")
6️⃣6️⃣ Check if a List is Sorted
nums = list(map(int, input("Enter numbers: ").split()))
print("Sorted" if nums == sorted(nums) else "Not Sorted")
6️⃣7️⃣ Rotate a List Left by K Positions
nums = list(map(int, input("Enter numbers: ").split()))
k = int(input("Enter rotation count: ")) % len(nums)
print(f"Rotated List: {nums[k:] + nums[:k]}")
6️⃣8️⃣ Find Common Elements in Two Lists
list1 = set(map(int, input("Enter first list: ").split()))
list2 = set(map(int, input("Enter second list: ").split()))
print(f"Common Elements: {list(list1 & list2)}")
6️⃣9️⃣ Find Union of Two Lists
list1 = set(map(int, input("Enter first list: ").split()))
list2 = set(map(int, input("Enter second list: ").split()))
print(f"Union: {list(list1 | list2)}")
7️⃣0️⃣ Find Intersection of Two Lists
list1 = set(map(int, input("Enter first list: ").split()))
list2 = set(map(int, input("Enter second list: ").split()))
print(f"Intersection: {list(list1 & list2)}")
7️⃣1️⃣ Find the Difference Between Two Lists
list1 = set(map(int, input("Enter first list: ").split()))
list2 = set(map(int, input("Enter second list: ").split()))
print(f"Difference: {list(list1 - list2)}")
7️⃣2️⃣ Convert a List to a String
words = input("Enter words: ").split()
print(" ".join(words))
7️⃣3️⃣ Reverse a List
nums = list(map(int, input("Enter numbers: ").split()))
print(f"Reversed List: {nums[::-1]}")
7️⃣4️⃣ Flatten a Nested List
nested = [[1, 2], [3, 4, 5], [6]]
flat_list = [item for sublist in nested for item in sublist]
print(f"Flattened List: {flat_list}")
7️⃣5️⃣ Find the Frequency of Each Element in a List
from collections import Counter
nums = list(map(int, input("Enter numbers: ").split()))
print(dict(Counter(nums)))
7️⃣6️⃣ Find the Largest Word in a Sentence
words = input("Enter a sentence: ").split()
print(f"Largest Word: {max(words, key=len)}")
7️⃣7️⃣ Count Words in a Sentence
sentence = input("Enter a sentence: ")
print(f"Word Count: {len(sentence.split())}")
7️⃣8️⃣ Reverse Words in a Sentence
sentence = input("Enter a sentence: ")
print(" ".join(sentence.split()[::-1]))
7️⃣9️⃣ Check if a String Contains a Substring
text = input("Enter a string: ")
substring = input("Enter substring: ")
print("Found" if substring in text else "Not Found")
8️⃣0️⃣ Convert a String to Uppercase
text = input("Enter a string: ")
print(text.upper())
8️⃣1️⃣ Convert a String to Lowercase
text = input("Enter a string: ")
print(text.lower())
8️⃣2️⃣ Swap Case of a String
text = input("Enter a string: ")
print(text.swapcase())
8️⃣3️⃣ Title Case a String
text = input("Enter a string: ")
print(text.title())
8️⃣4️⃣ Remove Punctuation from a String
import string
text = input("Enter a string: ")
print(text.translate(str.maketrans('', '', string.punctuation)))
8️⃣5️⃣ Find the Length of a String
text = input("Enter a string: ")
print(len(text))
8️⃣6️⃣ Replace a Word in a String
text = input("Enter a sentence: ")
old, new = input("Enter old and new word: ").split()
print(text.replace(old, new))
8️⃣7️⃣ Check if a String is Alphanumeric
text = input("Enter a string: ")
print("Alphanumeric" if text.isalnum() else "Not Alphanumeric")
8️⃣8️⃣ Sort Words in a Sentence Alphabetically
text = input("Enter a sentence: ")
print(" ".join(sorted(text.split())))
8️⃣9️⃣ Check if a String Contains Only Digits
text = input("Enter a string: ")
print("Contains Only Digits" if text.isdigit() else "Not Only Digits")
9️⃣0️⃣ Find Common Characters in Two Strings
str1 = set(input("Enter first string: "))
str2 = set(input("Enter second string: "))
print(f"Common Characters: {''.join(str1 & str2)}")
9️⃣1️⃣ Remove Spaces from a String
text = input("Enter a string: ")
print(text.replace(" ", ""))
9️⃣2️⃣ Reverse Each Word in a Sentence
text = input("Enter a sentence: ")
print(" ".join(word[::-1] for word in text.split()))
9️⃣3️⃣ Find the Most Frequent Character in a String
from collections import Counter
text = input("Enter a string: ")
print(f"Most Frequent Character: {Counter(text).most_common(1)[0][0]}")
9️⃣4️⃣ Count Upper and Lowercase Letters
text = input("Enter a string: ")
upper = sum(1 for c in text if c.isupper())
lower = sum(1 for c in text if c.islower())
print(f"Uppercase: {upper}, Lowercase: {lower}")
9️⃣5️⃣ Check if a String is an Anagram
str1, str2 = input("Enter two strings: ").split()
print("Anagram" if sorted(str1) == sorted(str2) else "Not Anagram")
9️⃣6️⃣ Print Even Numbers in a List
nums = list(map(int, input("Enter numbers: ").split()))
print([n for n in nums if n % 2 == 0])
9️⃣7️⃣ Print Odd Numbers in a List
nums = list(map(int, input("Enter numbers: ").split()))
print([n for n in nums if n % 2 != 0])
9️⃣8️⃣ Find the Median of a List
nums = sorted(map(int, input("Enter numbers: ").split()))
n = len(nums)
print(f"Median: {(nums[n//2] if n % 2 else (nums[n//2 - 1] + nums[n//2]) / 2)}")
9️⃣9️⃣ Find the Mode of a List
from statistics import mode
nums = list(map(int, input("Enter numbers: ").split()))
print(f"Mode: {mode(nums)}")
🔟0️⃣ Generate a Random Number
import random
print(f"Random Number: {random.randint(1, 100)}")
That’s all 100 Python examples! 🚀🐍 Let me know if you need more! Just Start whatsapp chat😊